Views A view is a logical representation of physical columns from one or multiple tables. A view looks and acts like a table, but it really isn't a physical table that resides on disk. Referred to by Informix as a virtual table, a view is a great way to present specific information to specific users, without placing an entire table's data in the open or keeping multiple versions of the data for different groups of users. Figure 16.1 shows how users view data from single or multiple tables through a view. Views provide a means to restrict certain columns from users. Sometimes tables contain tons of information that is useful to only a small segment of users. Most of the time, only one or two columns are actually accessed. A view can show and allow access to those few columns. This method makes the tables seem less overwhelming to users who don't fully understand all the data columns. Some data contained in a table may be sensitive to specific users for legal or moral reasons. You can use a view to allow users access to some of the data contained in sensitive tables while restricting access to other columns. For example, an employee table might contain information on each employee's address, phone number, who to notify in case of an emergency, and salary. Obviously, employees should not have access to other employees' salaries, but contact information could be important. Views can represent derived values as easily as they can show stored column data. Any computations allowed by SQL can be represented in a view with a virtual column. For example, you can create a current balance column in a view to represent the sum of all current orders, subtracting any payments received. Every time the database makes an order or payment, the balance column in the view is updated. You can represent derived data values within a view. By using the columns from one or more tables, the data placed in a view can be a derived value by using additions, subtraction, or any other mathematical function. For example, you can create a view showing the items, quantity, and value of orders placed by the customer Joe's Pizza, where the value is equal to the quantity multiplied against the price. To create a view, the user attempting the create must have at least select privileges on all the tables with columns that are represented in the view. The two parts to the view creation statement are view naming and column selection. A view's name must be a unique name of up to 18 characters. This name is used to access the view after it is created: CREATE VIEW view_name AS To assign columns to a view, use a standard SQL SELECT statement. The keywords ORDER BY and UNION are not allowed within a view's select statement, but all other select keywords are allowed. Data type values are automatically inherited from the original columns to the new view columns. The names of the columns can also be inherited to the view unless specified as something different. When creating a virtual column, you must specify a name for the new column. When a column name is specified, the CREATE VIEW statement requires that all column names be specified, regardless of whether the names are different: CREATE VIEW view_name (column list) AS SELECT columns FROM tables The first example sets up a standard view for the addresses of all employees who are currently employed full-time. No columns are renamed, so no column list is required. CREATE VIEW empl_address AS SELECT name, street, city, zip FROM employee_info WHERE current = Y AND work_status = F The next create sets up a view for all customers' ordering and payment activity. A virtual column is also created. You must list all columns because the virtual column needs a name. This view also joins two tables to retrieve the information: CREATE VIEW customer_bal (cust_id, last_order, last_payment, current_balance) AS SELECT cust_id, total_order, total_payment, total_order - total_payment FROM order_table, payment_table WHERE order_table.cust_id = payment_table.cust_id You can use other views' columns to build other views. The next create example determines the total outstanding balance owed to the company from the customer_view view. You can also use aggregate SQL commands to create virtual columns. Aggregate commands include SUM, MIN, MAX, AVG, and COUNT. The SUM command is used to add all the balances together: CREATE VIEW total_balance (balance) AS SELECT SUM(current_balance) FROM customer_view The next create example sets up a view on all the column data related to specific rows. All sales made by salesperson 12, Joe, are listed in the view: CREATE VIEW joes_sales AS SELECT * FROM sales WHERE sales_person = 12 The creator of a view is considered the owner; owners and DBA-level users can grant and revoke access to the view to other users. You can restrict access to an entire table but give users access to the table's data through a view. This forces the users to use the view to access the data. To restrict standard users from accessing the entire employee table but still allow them to access their addresses, you use the following example: REVOKE ALL ON employee_info; CREATE VIEW empl_address AS SELECT name, street, city, zip FROM employee_info; GRANT SELECT, UPDATE ON empl_address TO PUBLIC; Working with a view is just like accessing a table. Use the view name instead of the table name in all SQL commands. Some restrictions related to views are not found with individual tables. First, no indexes can be created on a view. Any table-related indexes are used when accessing the indexed data through a view. Any columns or tables used in a view must be present. If a table or view is dropped, any views that use the table or column are also dropped. Views that contain joins or aggregates can be accessed only with SELECT statements because a join or aggregate view takes different data items from different places and makes it look like it's all from one place. Informix cannot determine how a change to data in a view relates back to the original tables. Almost the same situation applies to virtual columns; because virtual columns are derived from multiple data sources, it is impossible to insert or update that value in a view. It is possible to delete the row from a view that contains a virtual column because Informix can trace back to the original column and keys. As mentioned in the previous discussion on creating views, you can create a view with information related to a specific data value or row. As in the joes_sales example, a view can contain a subset of a table or table's data. The joes_sales example showed the following code: CREATE VIEW joes_sales (sales_person, customer, sub_length, price) AS SELECT * FROM sales WHERE sales_person = 12; If this view is available for Joe to use, he might want to insert his new sales directly through this view rather than use the entire sales table. If Joe sells newspaper subscriptions and he makes a sale of a one month subscription to Mary Jones at $9.99, this information is placed in the sales table through the joes_sales view: INSERT INTO joes_sales VALUES (12, "Mary Jones", 1, 9.99); If Joe makes a mistake and uses the wrong sales_person number, his sale is credited to someone else: INSERT INTO joes_sales VALUES (11, "Mary Jones", 1, 9.99); Although he uses joes_sales, the insert for sales_person 11, Jake, succeeds back to the sales table. Joe can check his view: SELECT * FROM joes_sales; The entry for 11 does not show up because the view is limited to sales_person 12. Jake can see the entry if he has his own view: SELECT * FROM jakes_sales; Users with direct access to the sales table can also see the entry: SELECT * FROM sales; To prevent this problem, use the keyword WITH CHECK OPTION when creating the view. The WITH CHECK OPTION allows inserts, updates, and deletes to occur only when they meet the view select criteria: CREATE VIEW joes_sales (sales_person, customer, sub_length, price) AS SELECT * FROM sales WHERE sales_person = 12 WITH CHECK OPTION; When Joe tries to insert his sales with the wrong sales_person number, he receives an error message. An owner of the view or a DBA can drop an existing view. When you drop a view, you do not lose the data, columns, and tables; only the view to that data is gone. The data still resides in the underlying tables and columns of the database. On the other hand, if the actual tables and columns are dropped, any views that use those tables and columns are automatically dropped. In views such as joes_sales, if Joe has no sales in the sales table, the view continues to exist, but it contains zero rows. To drop a view, use the DROP VIEW command: DROP VIEW view_name; The following example uses DROP VIEW: DROP VIEW joes_sales; You cannot use an ALTER to change the layout of a view. If you need to change a view layout, you must drop and re-create the view with the new layout. To verify the current view layouts, use the sysviews and sysdepends system tables. The sysviews system table contains the actual CREATE VIEW statement originally entered. To see all the views currently in the database server, use SELECT * FROM sysviews; The sysdepends system table contains information on each view and the tables or other views that provide the data that makes up the original view. To see all the dependencies each view contains, use SELECT * FROM sysdepends; When a view is dropped, its information no longer resides in the sysviews or sysdepends tables. It is a good idea to save a copy of the preceding queries as a backup listing of all the views to use as a reference when creating or re-creating a view.
Still Reading Commercial Emails For Free? Receive Emails On Topics That Interests You And Get Paid For It! Get $10 Just to signup! Click Here

Friday, October 24, 2008
Informix Views
Labels: Informix
Informix Functions
Stored Procedures and Triggers Stored procedures and triggers are two essential functions that make a successful database server and also a successful database management system. Not only do stored procedures offer a viable means to reduce processing and network traffic, but they also help remove general and repetitive functionality from the different client applications. Stored procedures are considered separate database entities, and because they are separate, users must have the appropriate privileges to create, edit, and execute them. What is nice about stored procedures is that they can have access to specific areas of the database that users are not able to see. However, these same users might have the ability to run the stored procedure, which in turn performs specific functions in restricted areas. Therefore, the stored procedure enables users to go into restricted areas, but does not let them have full access to run wild. For example, a table contains all employees' salary and bonus information. A stored procedure is executed when a user enters information about a sale that earned commission. The stored procedure checks to see whether it's a valid commission, and then adds that amount to the appropriate person's salary. The user has no access to the table that contains the salary information, and if he or she tried to add the commission to the table without using the stored procedure, or perform any other activity on the table, it would fail. Triggers provide the means to automatically process a task when other events occur in the database, such as specific data access or creation. By combining triggers with stored procedures, you can build a library of processes to manage data security and auditing. Chapter 14, "Managing Data with Stored Procedures and Triggers," describes in detail how to use stored procedures and triggers to manage security.
Labels: Informix
Informix Privileges
Three levels of data-related security keep database users (users who must have some type of access to data in the database) from accessing specific data items. These levels are database, table, and column. All users must have access to a database to use data within a server. The three database-level privileges are connect, resource, and DBA. Table 16.1 shows the different authority levels associated with each privilege. Table 16.1. Database-level privileges. Privileges Connect Resource DBA Select, insert, update, delete, use temporary tables, and use views. Yes Yes Yes Create, alter, drop, and index own tables. No Yes Yes Grant, revoke, drop other owned tables, and start and stop server. No No Yes Connect The minimum database-level privilege is the connect level. Users with connect can perform select, insert, update, and delete statements, run stored procedures against tables, create views on tables, and create temporary tables with or without indexes. Resource Users with resource privileges have all the privileges of connect users. They also have the added ability to create, alter, and drop their own tables and place indexes on these tables. DBA The creator and owner of a database is automatically given DBA privileges. A DBA has the same privileges as the connect and resource users with added abilities. The added abilities include granting and revoking connect, resource, and DBA privileges to and from other users, and dropping and altering other users' tables and views. Users with DBA privilege can also drop, start, stop, and recover the database. Granting and Revoking Database-Level Privileges The user who creates the database is automatically given DBA privileges, which is the only level that can perform grants and revokes. The first DBA can create other DBAs with a grant statement in SQL. A grant gives authority to specific users at whatever level you choose. The DBA can also use a revoke to remove or lower the authority. Informix has a keyword called PUBLIC that represents all users who access the database server. To specify users, use their UNIX IDs. You can specify a list of users by separating each UNIX ID with a comma. The database to which users get access is the database to which the DBA is connected when running the SQL to perform the grant. If the database server has multiple databases, the DBA must perform a grant for each database to provide access to them all. If the user is allowed access to only one of the available databases, you perform the grant within only that specific database when it is open. To grant connect privileges, use this syntax: GRANT CONNECT TO PUBLIC; GRANT CONNECT TO user1; GRANT CONNECT TO usera,userb,userc; To revoke connect privileges, use this syntax: REVOKE CONNECT FROM PUBLIC; REVOKE CONNECT FROM user1; REVOKE CONNECT FROM usera,userb,userc; To grant resource privileges, use this syntax: GRANT RESOURCE TO PUBLIC; GRANT RESOURCE TO user1; GRANT RESOURCE TO usera,userb,userc; To revoke resource privileges, use this syntax: REVOKE RESOURCE FROM PUBLIC; REVOKE RESOURCE FROM user1; REVOKE RESOURCE FROM usera,userb,userc; To grant DBA privileges, use this syntax: GRANT DBA TO user1; GRANT DBA TO usera,userb,userc; To revoke DBA privileges, use this syntax: REVOKE DBA FROM user1; REVOKE DBA FROM usera,userb,userc; It is not a good idea to grant DBA privileges to PUBLIC. Imagine giving hundreds of users the ability to drop the database! When initially granting privileges, remember to grant only connect or resource levels to PUBLIC. Table-Level and Column-Level Privileges When a user has access to a database, the DBA can limit access to specific tables and columns within tables. The creator of the table or any resource-level or DBA-level user can create tables. That owner or any DBA can grant table-level privileges to other users for that table. A total of eight keywords provide different table-level privileges: insert, delete, select, update, references, index, alter, and all. Insert Granting insert privileges allows users to add new data to the table. Revoking that privilege stops users from adding data to the table. GRANT INSERT ON customer_table TO user1; REVOKE INSERT ON customer_table FROM PUBLIC; Delete Granting delete privileges allows users to remove data from a table. Revoking that privilege stops users from removing data from the table. GRANT DELETE ON customer_table TO user1; REVOKE DELETE ON customer_table FROM PUBLIC; Select Select privileges can be granted at the table level or at specific column levels. Users can have the ability to query an entire row in the table or just specific fields. In the first example, user1 can look at any column or any row of the customer_table. The second grant only allows PUBLIC to query only the customer_id and balance columns of the customer_table. You can revoke privileges in the same way. GRANT SELECT ON customer_table TO user1; GRANT SELECT (customer_id, balance) ON customer_table TO PUBLIC; REVOKE SELECT ON customer_table FROM user3; REVOKE SELECT (customer_id, balance) ON customer_table FROM user4; Update You can grant update privileges at the table level or specific column levels. Users can have the ability to change an entire row in the table or just specific fields. In the first example, user1 can update any column or any row of the customer_table. The second grant allows PUBLIC to update only the customer_id and balance columns of the customer_table. You can revoke privileges in the same way. GRANT UPDATE ON customer_table TO user1; GRANT UPDATE (customer_id, balance) ON customer_table TO PUBLIC; REVOKE UPDATE ON customer_table FROM user3; REVOKE UPDATE (customer_id, balance) ON customer_table FROM user4; References You can grant users the ability to force referential constraints on the entire row or specific columns of a table. The user must be a resource database-level user before the references privilege works. Referential constraints perform tasks such as cascading deletes or any other task that relies on how columns relate to other columns. GRANT REFERENCES ON customer_table TO user1; GRANT REFERENCES (customer_id, balance) ON customer_table TO PUBLIC; REVOKE REFERENCES ON customer_table FROM user3; REVOKE REFERENCES (customer_id, balance) ON customer_table FROM user4; Index The index privilege grants users the ability to create and drop indexes related to a table. Users must have the resource privilege in combination with the index privilege. Users with connect cannot create an index, even if they have the index privilege. There is no column-level privilege because indexes are built on all table rows. GRANT INDEX ON customer_table TO user1; REVOKE INDEX ON customer_table FROM user3; Alter The alter privilege allows users to change the layout of the columns within the table. Users with alter can add, delete, and change columns and the column data types. Only users with knowledge of the database system and how to protect it should have this privilege. This privilege is almost as high-level as DBA. Alter applies only to the table level. GRANT ALTER ON customer_table TO user1; REVOKE ALTER ON customer_table FROM user3; All The keyword all provides all table and column privileges to users. Using the all keyword grants or revokes any table privileges that the user might have. GRANT ALL ON customer_table TO user1; REVOKE ALL ON customer_table FROM user2; Combinations You can grant or revoke different combinations of table and column privileges in one command. Place the privileges in any sequence, separated by a comma, after the grant or revoke keyword. GRANT INSERT, DELETE, UPDATE ON customer_table TO PUBLIC; GRANT SELECT, UPDATE (customer_id, balance) ON customer_table TO user2; REVOKE INDEX, ALTER ON customer_table FROM user1; You can also combine table-level and column-level privileges in one statement. Column-level privileges use the specified columns, and table-level privileges use the specified table. GRANT INSERT, DELETE, SELECT, UPDATE (customer_id, balance) ON customer_table TO user2; REVOKE INDEX, SELECT, ALTER (customer_id, balance) ON customer_table FROM user3; Other Keywords You can use two other keywords in conjunction with the GRANT command. The first is the WITH GRANT OPTION keyword. When combined with the GRANT command, the user receiving the privileges can also grant the same privileges to other users. In the following example, user1 not only has insert, delete, select and update privileges on customer_table, but he or she can also grant any or all of these privileges to other users. GRANT INSERT, DELETE, SELECT, UPDATE ON customer_table TO user1 WITH GRANT OPTION; If user1 has one or all of the privileges revoked, all the users that user1 granted privileges to will also have the same privileges revoked. The other keyword used with grant is the AS keyword. The AS keyword allows you to perform a grant as if another user performs the grant. This sets up the situation described previously; if the grantor is revoked, all the users granted by that user are also revoked. Continuing with the preceding example, user1 was given insert, delete, select, and update privileges on customer_table and the right to grant these privileges. A DBA, the owner of the table, or the user that granted user1 the privileges could then grant as user1 to other users: GRANT INSERT, DELETE, SELECT, UPDATE ON customer_table TO user2, user3, user4, user5 AS user1; Now user1 through user5 have the same privileges. To revoke the privileges on all five users, just revoke user1: REVOKE ALL ON customer_table FROM user1; Security is an important issue with database creators, owners, providers, and users. Not only does security provide a means of keeping the data safe and intact from loss, but it also goes another level by keeping the content of the data safe and secure from misuse or abuse. You can achieve both levels of database security by using GRANT and REVOKE statements to set privileges at the database, table, and column levels. Setting different types of users also separates users responsible for managing the database, DBAs, and normal users. You can use stored procedures and triggers to audit how and when data is used or changed and also restrict access to data. You can set up a stored procedure to perform a task on data, and only privileged users can access that stored procedure to perform the task. Another way of restricting how users access data is through views. You can use a view to force users to perform tasks on a subset of the actual data, rather than access the entire database. Finally, you can use the operating system procedures to lock users out of the database and the entire system. Client applications should build in specific logon processes to allow only privileged users into the database server.
Labels: Informix
AIX Commands
COMMAND PARAMETER DESCRIPTION bootlist -m normal <dev1> <dev2> Alters the list of boot devices (or the ordering of these devices in the list available to the system) bosboot -a -ad /dev/rnt<x> -b <loc of bootimage> Create a boot image on the default boot device Create a boot image at location and send to tape cfgmgr Configures devices by running the programs in /etc/methods directory. chcons Redirects the system console to device or file, effective next startup chdev -l <device> -a<params> Changes a device's characteristics. chdisp Changes the display used by the low-function terminal (LFT subsystem. chfont Changes the default font selected at boot time. chfs -a size=+<blocks> /<FS> Changes attributes of a file system. This one will increase the Filesystem . chgroup Users=x,y,z <grp name> Changes attributes for groups. chlv -n <nlvname> <olvname> Rename logical volume from olvname to nlvname chps -s<no. pps> <ps name> Changes attributes of a paging space. chpv Changes the characteristics of a physical volume in a volume group. chque -q<q name> -a'host = x' Changes the queue name. chssys Changes a subsystem definition in the subsystem object class. chtcb Changes or queries the trusted computing base attribute of a file. chtz. Changes the system time zone information chuser rlogin=true <username> Changes attributes for the specified user. chvfs Changes entries in the /etc/vfs file. chvg Sets the characteristics of a volume group. crfs -v <fstype> (jfs) -d <lvname> -m <mount point> -Ayes Create a Filesystem on a pre-defined logical volume( which will automount at boot!) crfs -v <fstype> (jfs) -g <vgname> -a size='<no blocks>' -m <mount point> -Ayes Create a Filesystem and its logical volume (uses default lvname) crvfs Creates entries in the /etc/vfs file. exportvg None Export a volume group grpck -n ALL Verifies the correctness of a group definition. hvirprt Changes the attribute values of a virtual printer. importvg -y <vgname> <hdisk x> Import a Volume Group onto hdisk x lpstat List print queues lsallq Lists the names of all configured queues. lsallqdev Lists all configured printer and plotter queue device names within a specified queue. lsattr -El <devname> List detailed configuration for a device lscfg List all configured devices -v List in detail -l scsi* List all scsi IO devices lsdev -Cc tape List configured tape devices -Cc disk List configured disks on devices -C -s scsi -H List all defined scsi devices lsdisp Lists the displays currently available on the system. lsfont Lists the fonts available for use by the display. lsfs -l List all filesystems in /etc/filesystems quering the LVM descriptor area and superblocks lsfs Displays the characteristics of file systems. lsgroup ALL Displays the attributes of groups.(checks NIS aswell!) lsitab -a Lists the records in the /etc/inittab file. lskbd Lists the keyboard maps currently available to the low-function terminal (LFT subsystem. lslicense Displays the number of fixed licenses and the status of floating licensing. lslpp -l List all installed software lslv <lvname> List configuration details of a given Logical Volume -l <lvname> List further configuration details of a given disk lsprtsv -ch Shows print service information stored in the database. lsps -a List pagaing space lspv None List configured disks <hdisk> List configuration details of a given disk -l <hdisk> List Logical Volumes/Filesystems on a given disk lsque Displays the queue stanza name. lsquedev Displays the device stanza name. lssrc -a List all subsystems lsuser Displays attributes of user accounts. lsvfs Lists entries in the /etc/vfs file. lsvg None List configured Volume groups <vgname> List configuration details of a given Volume group -l <vgname> List Logical Volumes/Filesystems on a given Volume Group mkcatdefs Preprocesses a message source file. mkdev Adds a device to the system. mkfont Adds the font code associated with a display to the system. mkfontdir Creates a fonts.dir file from a directory of font files. mkgroup Creates a new group. mkitab Makes records in the /etc/inittab file. mklv -y <lvname> <vgname> <no of pps> Create a Logical Volume mklv Creates a logical volume. mklvcopy Adds copies to a logical volume. mknamsv Configures TCP/IP-based name service on a host for a client. mknotify Adds a notify method definition to the notify object class. mkprtsv Configures TCP/IP-based print service on a host. mkps Add an additional paging space to the system. mkque Adds a printer queue to the system. mkquedev Adds a printer queue device to the system. mkserver Adds a subserver definition to the subserver object class. mkssys Adds a subsystem definition to the subsystem object class. mksysb. Backs up mounted file systems in the rootvg volume group for subsequent reinstallation mkszfile. Records size of mounted file systems in the rootvg volume group for reinstallation mktcpip Sets the required values for starting TCP/IP on a host. mkuser Creates a new user account. mkuser.sys Customizes a new user account. Mkvg -y <vgname> -s <ppsize> <hdisk x> Create a Volume Group on hdisk x mkvg Creates a volume group. mkvirprt Makes a virtual printer. odmadd Adds objects to created object classes. odmchange Changes the contents of a selected object in the specified object class. odmcreate Produces the .c (source and .h (include files necessary for ODM application development and creates empty objectclasses. odmdelete Deletes selected objects from a specified object class. odmdrop Removes an object class. odmget Retrieves objects from the specified object classes and places them into an odmadd input file. odmshow Displays an object class definition on the screen. pwdck Verifies the correctness of local authentication information. redefinevg Redefines the set of physical volumes of the given volume group in the device configuration database. reducevg Removes physical volumes from a volume group. When all physical volumes are removed from the volume group, the volume group is deleted. reorgvg Reorganizes the physical partition allocation for a volume group. restbase Restores customized information from the boot image. rmdel Removes a delta from a Source Code Control System (SCCS file. rmdev Removes a device from the system. rmf Removes folders and the messages they contain. rmfs Removes a file system. rmgroup Removes a group. rmitab Removes records in the /etc/inittab file. rmlv Removes logical volumes from a volume group. rmlvcopy Removes copies from a logical volume. rmm Removes messages. rmnamsv Unconfigures TCP/IP-based name service on a host. rmnotify Removes a notify method definition from the notify object class. rmprtsv Unconfigures a print service on a client or server machine. rmps Removes a paging space from the system. rmque Removes a printer queue from the system. rmquedev Removes a printer or plotter queue device from the system. rmserver Removes a subserver definition from the subserver object class. rmssys Removes a subsystem definition from the subsystem object class. rmuser Removes a user account. rmvfs Removes entries in the /etc/vfs file. rmvirprt Removes a virtual printer. runcat Pipes the output data from the mkcatdefs command to the gencat command. savebase Saves base customized device data in the ODM onto the boot device. startsrc -s <subsystem> Start a subsystem stopsrc -s <subsystem> Stop a subsystem syncvg Synchronizes logical volume copies that are not current. usrck Verifies the correctness of a user definition. varyoffvg <vgname> Takes a Volume Group offline varyoffvg Deactivates a volume group. varyonvg <vgname> Puts a Volume Group online varyonvg Activates a volume group.
Labels: IBM AIX
Travel Packages
Packages: Langkasuka Hotel |
Duration: Per Night |
Location:Langkawi |
80% of 90,000 Hotels worldwide available at lowest price guaranteed! |
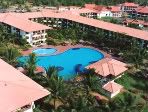